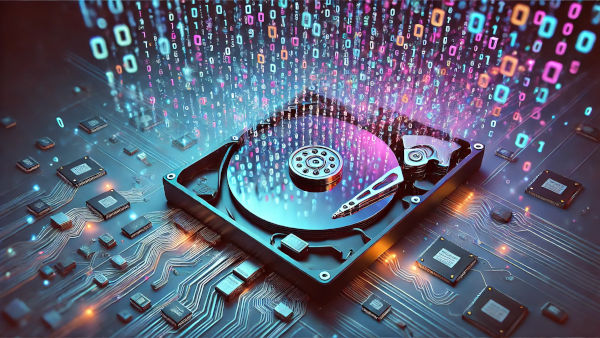
When I need to erase a hard drive, I like to fill it to the last byte with random data. My general process looks kinda like this:
- Delete all partitions on the drive.
- Create one large new partition that takes up the whole drive.
- Encrypt the partition (I usually use BitLocker with a 256 character random string password).
- Fill the entire drive with random data so there’s nothing left to recover.
- Delete the big partition on the drive again.
I wrote this PowerShell script to automate that last step. It simply fills a target drive with random data and, if you want, it also optionally runs SDelete (from Microsoft’s most excellent Sysinternals Suite) to overwrite any remaining slack space that may be left over at the end. Here are the basics of what it does…
- It scans your system for all drives but automatically excludes the system drive, boot drive, and any network drives.
- It shows you a list of available drives to choose from.
- Once you pick a drive, it fills all free space with random data using ~1GB files.
- When the drive gets close to full, it switches to smaller file sizes until there’s zero free space left.
- At the end, it asks if you want to run SDelete to securely wipe any remaining slack space.
- If you say yes, it downloads and runs SDelete automatically.
This script does NOT delete any of your existing files or partitions—it only overwrites free space to make sure previously deleted data can’t be recovered. If you run it on a drive with existing files, it won’t touch them. But it will completely fill the rest of the drive.
How to Run the Script
- Copy the script from the code window below and paste it into your favorite code editor (I like to use VS Code) , Powershell ISE, or just notepad. Then save it somewhere on your hard drive. (As with any script you download from the internet, make sure you read through the script and understand what its doing before actually running it – This is good advice in general)
- Start Windows Powershell (As Administrator).
- Start the script by typing
filldrive.ps1
and press enter.
If PowerShell blocks the script from running, enter the following command to allow the script to run.
“Set-ExecutionPolicy -Scope Process -ExecutionPolicy RemoteSigned -Force“
and then try running filldrive.ps1 again.
What to expect
- The script will quickly scan all drives on your system .
- It will show you only the drives that are safe to fill (ex NOT the system, boot, or network drives)
- You will be presented with a list of available drives (excluding your System, Boot, and Network drives)
- Pick the drive that you want to fill by typing the drive letter and pressing Enter.
- The script will give you a final warning and ask:“Are you sure you want to overwrite free space on drive X?”
- If you choose No, the script exits.
- If you choose Yes, the script begins the filling process
Overwriting the Drive
- A folder called FillDriveTemp is created on the target drive.
- The script fills the drive with ~1GB files filled with random data.
- When there is no longer enough space for 1Gb files, it switches to creating smaller files.
- This this step can take several hours to complete, depending on the drive’s size, type, and speed.
- You can keep using your computer while the script runs—just minimize the PowerShell window.
Optional: Running SDelete
- Once the drive is full, the script asks:“Do you want to run SDelete to overwrite any remaining free or slack space?”
- If you select No, the script exits.
- If you select Yes, the script downloads and runs SDelete, then exits when it’s done. (This is typically a quick operation)
What to Do After the Script Finishes
- If your intention was to wipe the entire drive, you can now delete the partition and move on.
- If you just wanted to securely erase the free space, delete the FillDriveTemp folder, and you’re done.
<#
SYNOPSIS
Overwrites all FREE SPACE on a selected drive with random data to prevent
the recovery of previously deleted data.
DESCRIPTION
This script securely overwrites all free space on a selected drive by:
- Displaying a list of available drives (excluding system, boot, and network drives).
- Filling the drive with truly random data until all free space on the drive is occupied.
- Optionally running SDelete after the fill process to overwrite any remaining slack space.
(If SDelete is not detected on the system, the script will prompt the user to download and run it.)
- Preventing operations on system, boot, and network drives for safety.
This process does NOT erase existing files or partitions. It only ensures
that previously deleted data cannot be recovered.
PARAMETER None
The script requires no parameters. It will prompt the user for input.
NOTES
File Name : FillDrive.ps1
Version : 25.2.20.01
Author : Charles Ostertag - ByteMaverick
Website : https://502tech.com
Creation Date : [11/23/2024]
Last Modified : [02/20/2025]
Purpose : Overwrite ALL FREE SPACE with random data to prevent recovery of previously deleted data.
Requirements : - Windows PowerShell
- Internet connection (for downloading SDelete - if needed)
- SDelete.exe (optional for additional secure overwrite of any slack space)
LICENSE
MIT License
Copyright (c) 2025 Charles Ostertag - ByteMaverick
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
THE ABOVE COPYRIGHT NOTICE AND THIS PERMISSION NOTICE SHALL BE INCLUDED IN
ALL COPIES OR SUBSTANTIAL PORTIONS OF THE SOFTWARE.
**Attribution is appreciated but not required. If you would like to give credit,
please mention:**
Charles Ostertag - ByteMaverick
Website: (https://502tech.com)
THE SOFTWARE IS PROVIDED "AS IS," WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES, OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS
IN THE SOFTWARE.
DISCLAIMER
**USE THIS SCRIPT AT YOUR OWN RISK.**
This script is designed to overwrite free space on a drive with random data
to prevent the recovery of previously deleted files and data. It does NOT erase existing files,
partitions, or the drive itself, but it will make previously deleted data unrecoverable.
SSD Drive Considerations:
This script performs extensive writes to the drive, which can cause excessive wear on SSDs over time due to write amplification.
While necessary for secure erasure, frequent use on SSDs can shorten their lifespan due to excessive write cycles.
BEFORE RUNNING THIS SCRIPT:
- **READ THROUGH THE SCRIPT CAREFULLY** Make sure you understand what it does, and how it does it.
- **BACK UP ANY IMPORTANT DATA** before running the script.
- **TEST IT ON A NON-CRITICAL SYSTEM** if you are unsure.
WARRANTY
This software is provided "as is," without warranty of any kind, express or implied, including but
not limited to any warranties of merchantability, fitness for a particular purpose, and noninfringement.
In no event shall the authors or copyright holders be liable for any claim, damages, or other liability,
whether in an action of contract, tort, or otherwise, arising from, out of, or in connection with the
software or the use or other dealings in the software.
USAGE
1. Run PowerShell as Administrator.
2. If script execution is restricted, allow running unsigned scripts:
Set-ExecutionPolicy -Scope Process -ExecutionPolicy RemoteSigned -Force
You can also run
powershell -NoProfile -ExecutionPolicy Bypass -File "filldrive.ps1"
3. Execute the script:
.\FillDrive.ps1
4. Follow the prompts to select a target drive and begin the overwrite process.
WARNING
- This script does NOT delete EXISTING files.
- It will overwrite ALL free space, making previously deleted data unrecoverable.
- It will COMPLETELY FILL THE TARGET DRIVE
- You should ensure backups exist before proceeding.
EXAMPLE
Run the script:
PS C:\> .\FillDrive.ps1
or
powershell -NoProfile -ExecutionPolicy Bypass -File "filldrive.ps1"
The script will display all available drives, prompt the user for selection, and
begin overwriting free space.
LINK
SDelete (Sysinternals):
https://docs.microsoft.com/en-us/sysinternals/downloads/sdelete
#>
##############################################################
####### OKAY, LET"S GET STARTED ########
##############################################################
# Display info - Display script information
clear
Write-Host " ByteMaverick's FillDrive Script"
Write-Host "This script will fill all unused space on a target drive with randomly generated"
Write-Host "data and then optionally use SDelete to securely erase any remaining slack space."
Write-Host "Note: Boot, System, and Network drives are not listed as possible targets"
Write-Host
Write-Host "Please enter a drive letter from the list below to fill"
Write-Host "with randomly generated data or press CTRL+C to exit."
Write-Host
#MARK: Function Definitions
# Function: Write-RandomDataToFile
# Purpose: Writes random data to a specified file until it reaches the desired size.
function Write-RandomDataToFile {
param (
[string]$FilePath, # The full path of the file to write to.
[long]$FileSize # The desired size of the file in bytes.
)
try {
# Define the buffer size (1 MB).
$bufferSize = 1048576
$buffer = New-Object Byte[] $bufferSize
# Initialize a cryptographic random number generator.
$rng = New-Object System.Security.Cryptography.RNGCryptoServiceProvider
$bytesWritten = 0
# Open a file stream to write data.
$fileStream = [System.IO.File]::Open($FilePath, [System.IO.FileMode]::Create, [System.IO.FileAccess]::Write, [System.IO.FileShare]::None)
# Loop until the desired file size is reached.
while ($bytesWritten -lt $FileSize) {
# Calculate the remaining bytes to write.
$remainingBytes = $FileSize - $bytesWritten
if ($remainingBytes -lt $bufferSize) {
$bytesToWrite = [int]$remainingBytes
} else {
$bytesToWrite = $bufferSize
}
# Generate random bytes and write them to the file.
$rng.GetBytes($buffer, 0, $bytesToWrite)
$fileStream.Write($buffer, 0, $bytesToWrite)
$bytesWritten += $bytesToWrite
# Display progress to the user.
$percentComplete = [Math]::Round(($bytesWritten / $FileSize) * 100, 2)
Write-Progress -Activity "Writing $FilePath" -Status "$percentComplete% Complete" -PercentComplete $percentComplete
}
# Close the file stream.
$fileStream.Close()
} catch {
Write-Host "Error writing to file ${FilePath}: $_"
}
}
#MARK: SDelete
# Purpose: Downloads SDelete if necessary and runs it to overwrite free or slack space on the target drive.
function Run-SDelete {
param (
[string]$DriveLetter # The drive letter to run SDelete on.
)
# Set the path for SDelete.exe in the TEMP directory.
$sdeletePath = "$env:TEMP\SDelete.exe"
# Check if SDelete.exe exists.
if (!(Test-Path $sdeletePath)) {
Write-Host "SDelete not found. Downloading SDelete..."
# Define the URL and path for the SDelete ZIP file.
$sdeleteUrl = "https://download.sysinternals.com/files/SDelete.zip"
$zipPath = "$env:TEMP\SDelete.zip"
try {
# Download SDelete.zip from the official Microsoft Sysinternals website.
Invoke-WebRequest -Uri $sdeleteUrl -OutFile $zipPath
# Extract SDelete.exe from the ZIP file.
$shellApp = New-Object -ComObject Shell.Application
$zipFile = $shellApp.NameSpace($zipPath)
$destinationFolder = $shellApp.NameSpace((Split-Path $sdeletePath))
$destinationFolder.CopyHere($zipFile.Items(), 0x10) # 0x10 = Do not display a progress dialog box
# Remove the downloaded ZIP file.
Remove-Item $zipPath -Force
Write-Host "SDelete downloaded and extracted successfully."
} catch {
Write-Host "Error downloading or extracting SDelete: $_"
return
}
} else {
Write-Host "SDelete found at $sdeletePath."
}
try {
# Execute SDelete to overwrite free or slack space.
Write-Host "Executing SDelete on drive $DriveLetter..."
& $sdeletePath -accepteula -p 1 -z $DriveLetter`:
Write-Host "SDelete completed successfully."
} catch {
Write-Host "Error running SDelete: $_"
}
}
#MARK: Drive Selection
# Get the system and boot drive letters.
$systemDriveLetter = [System.Environment]::GetEnvironmentVariable("SystemDrive").TrimEnd(":").ToUpper()
$bootDrive = Get-PSDrive -PSProvider FileSystem | Where-Object { $_.DisplayRoot -eq '' -and $_.Root -eq '\' }
if ($bootDrive) {
$bootDriveLetter = $bootDrive.Name.TrimEnd(":").ToUpper()
} else {
# If unable to determine the boot drive, default to system drive letter.
$bootDriveLetter = $systemDriveLetter
}
# Get all local fixed and removable drives excluding the system and boot drives.
$availableDrives = [System.IO.DriveInfo]::GetDrives() | Where-Object {
$_.IsReady -and # Drive is ready.
$_.DriveType -eq 'Fixed' -or $_.DriveType -eq 'Removable' -and # Include local fixed and removable drives.
($_.Name.TrimEnd('\').TrimEnd(':').ToUpper() -ne $systemDriveLetter) -and # Exclude system drive.
($_.Name.TrimEnd('\').TrimEnd(':').ToUpper() -ne $bootDriveLetter) # Exclude boot drive
}
# Check if there are any available drives to process.
if ($availableDrives.Count -eq 0) {
Write-Host "No suitable drives found to securely erase."
exit
}
# Display the list of available drives to the user, including labels.
Write-Host "Available drives to fill:"
foreach ($drive in $availableDrives) {
$driveLetterDisplay = $drive.Name.TrimEnd('\')
$driveSizeGB = [Math]::Round($drive.TotalSize / 1GB, 2)
$driveLabel = $drive.VolumeLabel
if ([string]::IsNullOrEmpty($driveLabel)) {
$driveLabel = "No Label"
}
Write-Host " - $driveLetterDisplay `"$driveLabel`" ($driveSizeGB GB)"
}
Write-Host
# MARK: Get User Input
# Prompt user for the target drive letter from the list above.
$driveLetter = Read-Host "Enter the letter of the drive you wish to fill from the list above"
# Validate and format the drive letter.
$driveLetter = $driveLetter.TrimEnd(":").ToUpper()
# Check if the selected drive is in the available drives list.
$targetDrive = $availableDrives | Where-Object { $_.Name -eq "$driveLetter`:\" }
if (!$targetDrive) {
Write-Host "Invalid drive selected or drive is not available."
exit
}
#MARK: Display warning
Write-Host
Write-Host "WARNING: You are about to fill drive $driveLetter with random data. All previously"
Write-Host "deleted data on this drive will be OVERWRITTEN and CANNOT be recovered."
$confirm = Read-Host "Are you sure you want to proceed? (Y/N)"
if ($confirm -ne 'Y' -and $confirm -ne 'y') {
Write-Host "Operation canceled."
exit
}
#MARK: Fill Drive
# Create a temporary directory on the target drive to store random data files.
$tempDirPath = Join-Path $targetDrive.RootDirectory.FullName "SecureEraseTemp"
if (!(Test-Path $tempDirPath)) {
New-Item -ItemType Directory -Path $tempDirPath | Out-Null
} else {
Write-Host "Temporary directory already exists: $tempDirPath"
}
# Initialize file counter.
$fileIndex = 1
# Loop to fill the drive with random data files until it's full.
try {
while ($true) {
# Recreate the DriveInfo object to get updated information.
$targetDrive = New-Object System.IO.DriveInfo("$driveLetter`:\")
# Get available free space on the drive.
$freeSpace = $targetDrive.AvailableFreeSpace
if ($freeSpace -le 0) {
Write-Host "Drive is full."
break
}
# Determine the file size to write (1 GB or the remaining free space).
if ($freeSpace -lt 1073741824) {
$fileSize = $freeSpace
} else {
$fileSize = 1073741824 # 1 GB
}
# Construct the full file path for the random data file.
$fileName = Join-Path $tempDirPath "RandomData$fileIndex.bin"
Write-Host "Creating file $fileName of size $fileSize bytes..."
# Write random data to the file.
Write-RandomDataToFile -FilePath $fileName -FileSize $fileSize
$fileIndex++
# Recreate the DriveInfo object again for updated info.
$targetDrive = New-Object System.IO.DriveInfo("$driveLetter`:\")
$freeSpace = $targetDrive.AvailableFreeSpace
if ($freeSpace -le 0) {
Write-Host "Drive is full."
break
}
}
} catch {
Write-Host "An error occurred: $_"
}
#MARK: Post-Processing
Write-Host
Write-Host "Drive $driveLetter has been filled with random data."
#MARK: SDelete - Ask if the user wants to run SDelete to overwrite any remaining free or slack space.
$sdeleteConfirm = Read-Host "Do you want to run SDelete to overwrite any remaining free or slack space? (Y/N)"
if ($sdeleteConfirm -eq 'Y' -or $sdeleteConfirm -eq 'y') {
Run-SDelete -DriveLetter $driveLetter
}
Write-Host
Write-Host "Secure erasure of drive $driveLetter is complete."
# All Done!
Below are some screenshots of the script being run so you know what to expect.



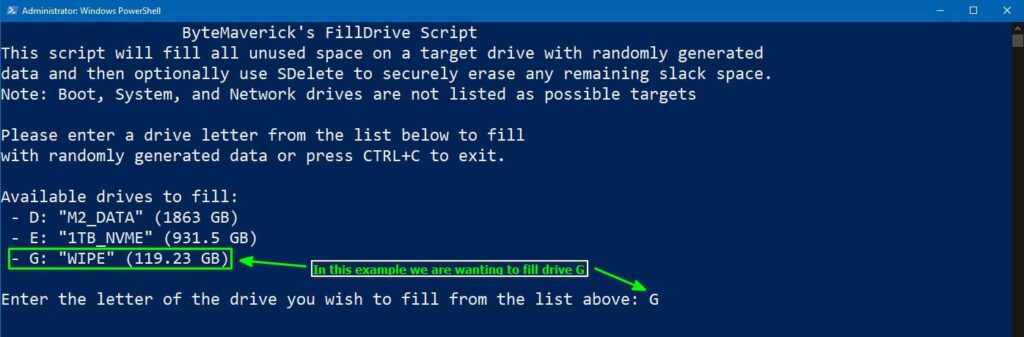
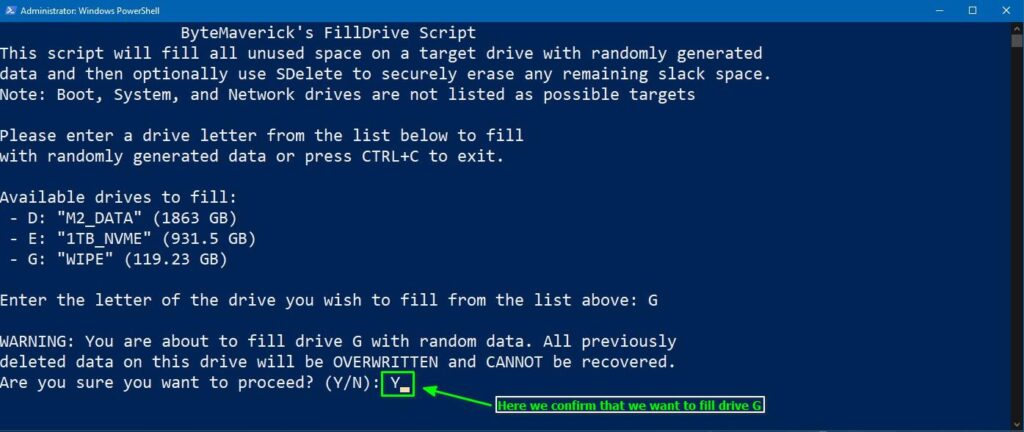
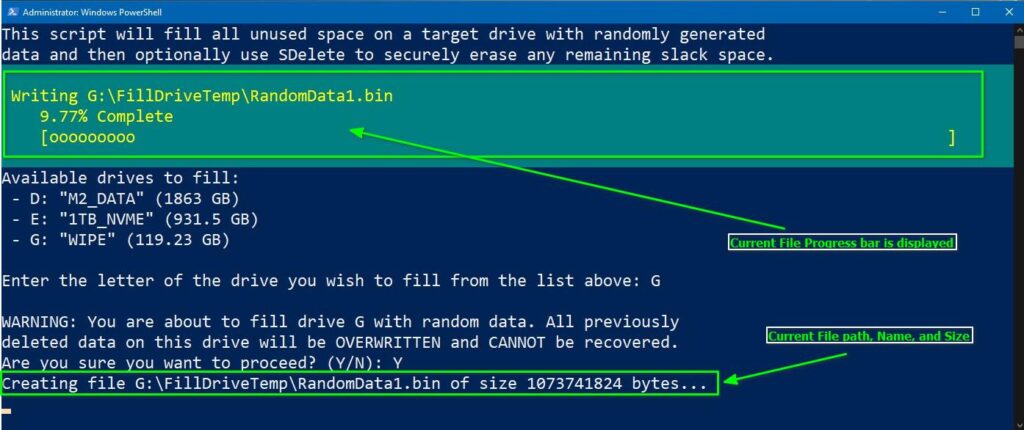
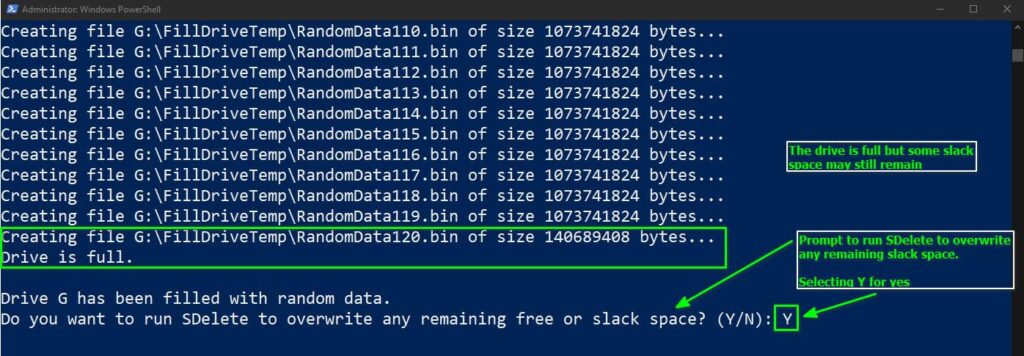
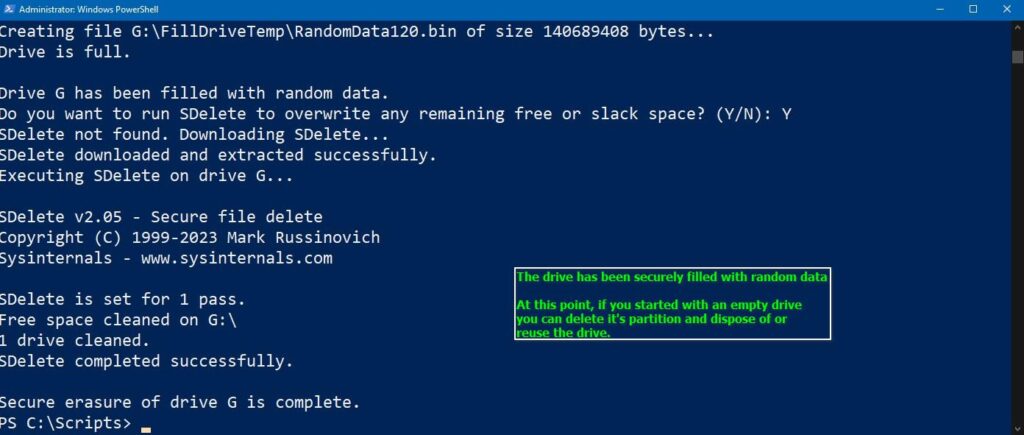
Thats it! I hope you enjoy the script. 😃